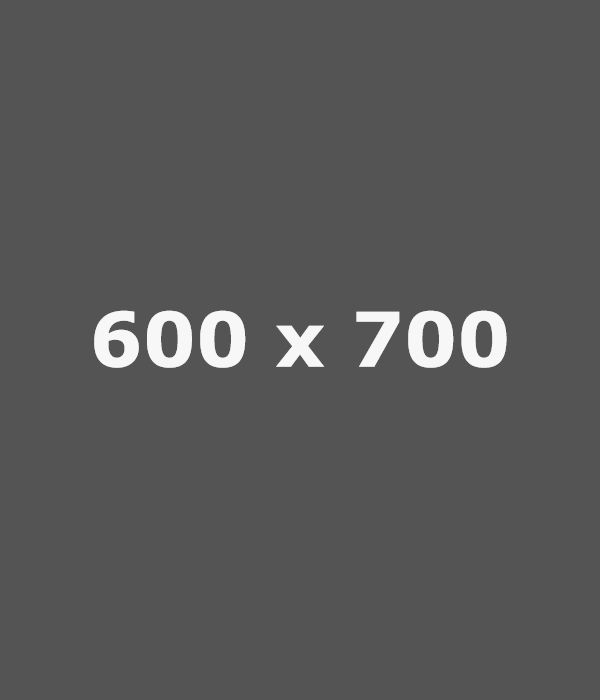
Name :
Nikhil Mittal
Email :
me@nikhilmittal.in
Address :
R1, Life Repuplic, PN, MH, IN
About Me :
Your friend & mentor !
© 2021 NM. Designd By Nikhil Mittal All Rights Reserved
In ASP.NET Core, middleware components handle the incoming requests and outgoing responses. Each middleware can decide to process a request, ignore it, or pass it along the pipeline. These components are executed in the same sequence in which they are added.
Previously, ASP.NET used HTTP modules and handlers for tasks like caching, authorization, and request handling. HTTP modules provided application-level services by hooking into lifecycle events, while handlers were responsible for generating responses. Modules and handlers were driven by application events, whereas middleware execution is based on their order in the pipeline.
Middleware in ASP.NET Core offers the same functionalities as modules and handlers and can integrate with frameworks like MVC. Middleware components execute sequentially as they are added, while HTTP handlers execute with each associated application event, and HTTP modules execute once to generate a response.
MIDDLEWARE ! How to Configure
In .NET Core, the methods Use, Map, and MapWhen are used to configure the middleware pipeline, however the usage is different in different scenarios.
We can add N number of app.Use methods.
The sequence of execution will be in the order in which they were added to the pipeline.
It also has the option to pass the request to the next component or to short-circuit the pipeline.
When middleware is written as an app.Use(……) method, a middleware delegate gets added to the application’s request pipeline.
When a request matches the specified path segment, only the middleware which is configured within Map gets executed.
E.G. based on Params
Example:
app.Use(async (context, next) =>
{
await context.Response.WriteAsync("Middleware Three</br>");
await next.Invoke();
});
app.Map("/branch1", (appBuilder) =>
{
appBuilder.Use(async (context, next) =>
{
await context.Response.WriteAsync("--Branch 1 - Middleware One</br>");
await next.Invoke();
});
appBuilder.Use(async (context, next) =>
{
await context.Response.WriteAsync("--Branch 1 - Middleware Two</br>");
await next.Invoke();
});
});
app.Run(async context =>
{
await context.Response.WriteAsync("end of the pipeline.");
});
BUILT IN MIDDLEWARES IN Dot Net Core
Comments
Leave your Comment here